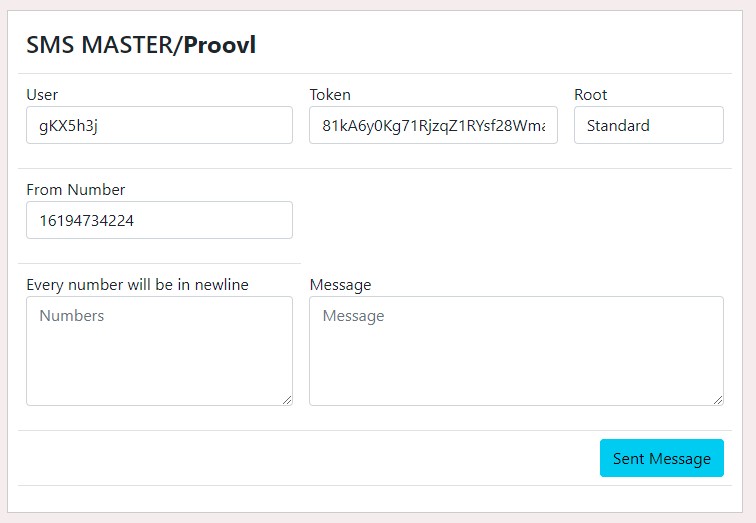
In this tutorial, we will see how to send bulk SMS using proovl SMS API in PHP follow the few simple steps to send bulk SMS
Step 1: Create the index.php
create index.php file and put the following code
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<link href="assets/style.css" rel="stylesheet" type="text/css"/>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link href="https://fonts.googleapis.com/css?family=Roboto" rel="stylesheet">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.1/dist/css/bootstrap.min.css" rel="stylesheet" crossorigin="anonymous">
</head>
<body>
<div class="container">
<div class="row">
<div class="col-2"></div>
<div class="col-8">
<div class="my-table">
<table class="table">
<tr>
<td colspan="5">
<h4>SMS MASTER/<strong>Proovl</strong></h4>
<div id="responce" class="badge bg-secondary"></div>
</td>
</tr>
<tr>
<td colspan="3">
<div class="mb-3">
<label>User</label>
<input type="text" id="user" placeholder="user" class="form-control" value="gKX5h3j">
</div>
</td>
<td>
<div class="mb-3">
<label>Token</label>
<input type="text" id="token" placeholder="Token" class="form-control" value="81kA6y0Kg71RjzqZ1RYsf28Wma3Lyann">
</div>
</td>
<td>
<div class="mb-3">
<label>Root</label>
<select class="form-control" id="route">
<option value="1">Standard</option>
<option value="2">Economy</option>
</select>
</div>
</td>
</tr>
<tr>
<td>
<div class="mb-3">
<label>From Number</label>
<input type="text" id="from" placeholder="From Number" class="form-control" value="16194734224">
</div>
</td>
</tr>
<tr>
<td colspan="3">
<div class="mb-3">
<label for="floatingTextarea">Every number will be in newline</label>
<textarea class="form-control" placeholder="Numbers" id="mobiles" rows="4"></textarea>
</div>
</td>
<td colspan="2">
<div class="mb-3">
<label for="floatingTextarea">Message</label>
<textarea class="form-control" placeholder="Message" id="message" rows="4"></textarea>
</div>
</td>
</tr>
<tr>
<td colspan="4"></td>
<td class="text-end">
<input type="button" id="submit-btn" value="Sent Message" class="btn btn-info" onclick="start_sendig();">
</td>
</tr>
</div>
</div>
<div class="col-2"></div>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js" type="text/javascript"></script>
<script src="assets/main.js" type="text/javascript"></script>
</body>
</html>
When you run the file it will ask you user, Token, root and From number
Step 2: multiple_number.php
This file contains actual request to send sms using curl
<?php
if (isset($_POST['numbers'])) {
$numbers = $_POST['numbers'];
$message = $_POST['message'];
$user = $_POST['user'];
$token = $_POST['token'];
$route = $_POST['route'];
$from = $_POST['from'];
$url = "https://www.proovl.com/api/send.php";
foreach (explode("+", $numbers) as $phone) {
$postData = array(
'user' => $user,
'token' => $token,
'route' => "$route",
'from' => "$from",
'to' => ltrim($phone, ','),
'text' => $message,
);
$ch = curl_init();
curl_setopt_array($ch, array(
CURLOPT_URL => $url,
CURLOPT_RETURNTRANSFER => TRUE,
CURLOPT_POST => TRUE,
CURLOPT_POSTFIELDS => $postData,
CURLOPT_FOLLOWLOCATION => TRUE
));
//Ignore SSL certificate verification
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 0);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, 0);
$response = curl_exec($ch);
if ($response) {
$data = array(
"response" => "success",
"current" => ltrim($phone, ',')
);
echo json_encode($data);
}
curl_close($ch);
}
}
Step 3: Create assets directories
Create assets directories and create two files main.js and style.css
main.js
function start_sendig() {
$('#responce').html("Sending to <span id='curent-number'></span>");
var mobiles = $('#mobiles').val().replace(/(?:\r\n|\r|\n)/g, ',');
var message = $('#message').val();
var path_uri = "multiple_number.php";
var number = mobiles.split('+');
var user = $('#user').val();
var token = $('#token').val();
var route = $('#route').val();
var from = $('#from').val();
$.ajax({
type: "POST",
url: path_uri,
data: {
numbers: number_loop(number),
message: message,
user: user,
token: token,
route: route,
from: from
},
success: function (data) {
console.log(data);
var json = $.parseJSON(data);
if (json[2] == "nexmo") {
if (json[1] == "0") {
$('#responce').html("Message Sent Successfully to " + json[0] + " !!");
}
} else if (json.response == "success") {
$('#responce').html("Message Sent Successfully!");
} else {
$('#responce').html("Error to Sent!");
}
}
});
}
var i = 0;
function numbers_loop(numbers) {
var number = numbers[i];
$("#curent-number").html("Sending please wait.....");
if (++i < numbers.len) {
setTimeout(start_sendig, 1000);
}
return number;
}
//upload xls
$('#upload_list').on('change', function () {
$('#outer-loader').show();
var file_data = $('#upload_list').prop('files')[0];
var form_data = new FormData();
form_data.append('file', file_data);
$.ajax({
url: 'uploadtxt.php', // point to server-side PHP script
dataType: 'text', // what to expect back from the PHP script, if anything
cache: false,
contentType: false,
processData: false,
data: form_data,
type: 'post',
success: function (data) {
$('#numbers').html(data);
}
});
});
style.css
/*
Created on : Sep 15, 2018, 2:39:58 PM
Author : Gajanand Rathor
*/
body{
background: #f3eded!important;
margin: 0;
padding: 0;
}
.my-table{
border: 1px solid #ccc;
padding: 10px;
background: #fff;
margin-top: 40px;
}
Still you are facing problem to send bulk SMS you can download zip file and extract .
Thanks..